Welcome to Part 2 of our tour through modern machine learning algorithms. In this part, we’ll cover methods for Dimensionality Reduction, further broken into Feature Selection and Feature Extraction. In general, these tasks are rarely performed in isolation. Instead, they’re often preprocessing steps to support other tasks.
If you missed Part 1, you can check it out here. It explains our methodology for categorization algorithms, and it covers the “Big 3” machine learning tasks:
- Regression
- Classification
- Clustering
In this part, we’ll cover:
We will also cover other tasks, such as Density Estimation and Anomaly Detection, in dedicated guides in the future.
The Curse of Dimensionality
In machine learning, “dimensionality” simply refers to the number of features (i.e. input variables) in your dataset.
When the number of features is very large relative to the number of observations in your dataset, certain algorithms struggle to train effective models. This is called the “Curse of Dimensionality,” and it’s especially relevant for clustering algorithms that rely on distance calculations.
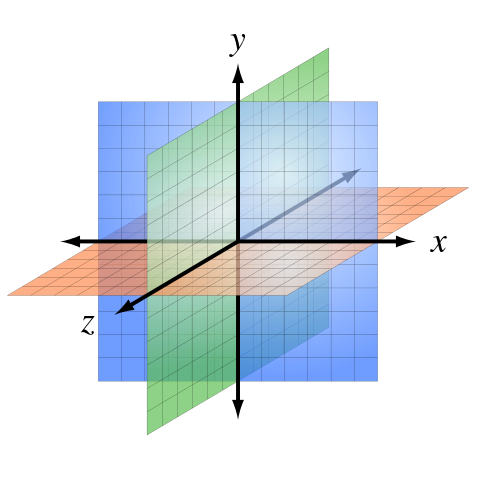
A Quora user has provided an excellent analogy for the Curse of Dimensionality, which we’ll borrow here:
Let’s say you have a straight line 100 yards long and you dropped a penny somewhere on it. It wouldn’t be too hard to find. You walk along the line and it takes two minutes.
Now let’s say you have a square 100 yards on each side and you dropped a penny somewhere on it. It would be pretty hard, like searching across two football fields stuck together. It could take days.
Now a cube 100 yards across. That’s like searching a 30-story building the size of a football stadium. Ugh.
The difficulty of searching through the space gets a lot harder as you have more dimensions.
In this guide, we’ll look at the two primary methods for reducing dimensionality: Feature Selection and Feature Extraction.
4. Feature Selection
Feature selection is for filtering irrelevant or redundant features from your dataset. The key difference between feature selection and extraction is that feature selection keeps a subset of the original features while feature extraction creates brand new ones.
To be clear, some supervised algorithms already have built-in feature selection, such as Regularized Regression and Random Forests. Typically, we recommend starting with these algorithms if they fit your task. They’re covered in Part 1: Modern Machine Learning Algorithms.
As a stand-alone task, feature selection can be unsupervised (e.g. Variance Thresholds) or supervised (e.g. Genetic Algorithms). You can also combine multiple methods if needed.
4.1. Variance Thresholds
Variance thresholds remove features whose values don’t change much from observation to observation (i.e. their variance falls below a threshold). These features provide little value.
For example, if you had a public health dataset where 96% of observations were for 35-year-old men, then the ‘Age’ and ‘Gender’ features can be eliminated without a major loss in information.
Because variance is dependent on scale, you should always normalize your features first.
- Strengths: Applying variance thresholds is based on solid intuition: features that don’t change much also don’t add much information. This is an easy and relatively safe way to reduce dimensionality at the start of your modeling process.
- Weaknesses: If your problem does require dimensionality reduction, applying variance thresholds is rarely sufficient. Furthermore, you must manually set or tune a variance threshold, which could be tricky. We recommend starting with a conservative (i.e. lower) threshold.
- Implementations: Python / R
4.2. Correlation Thresholds
Correlation thresholds remove features that are highly correlated with others (i.e. its values change very similarly to another’s). These features provide redundant information.
For example, if you had a real-estate dataset with ‘Floor Area (Sq. Ft.)’ and ‘Floor Area (Sq. Meters)’ as separate features, you can safely remove one of them.
Which one should you remove? Well, you’d first calculate all pair-wise correlations. Then, if the correlation between a pair of features is above a given threshold, you’d remove the one that has larger mean absolute correlation with other features.
- Strengths: Applying correlation thresholds is also based on solid intuition: similar features provide redundant information. Some algorithms are not robust to correlated features, so removing them can boost performance.
- Weaknesses: Again, you must manually set or tune a correlation threshold, which can be tricky to do. Plus, if you set your threshold too low, you risk dropping useful information. Whenever possible, we prefer algorithms with built-in feature selection over correlation thresholds. Even for algorithms without built-in feature selection, Principal Component Analysis (PCA) is often a better alternative.
- Implementations: Python / R
4.3. Genetic Algorithms (GA)
Genetic algorithms (GA) are a broad class of algorithms that can be adapted to different purposes. They are search algorithms that are inspired by evolutionary biology and natural selection, combining mutation and cross-over to efficiently traverse large solution spaces. Here’s a great intro to the intuition behind GA’s.
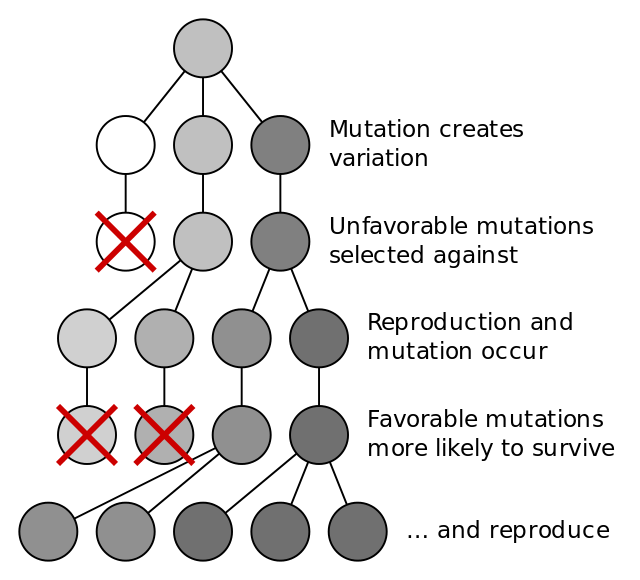
In machine learning, GA’s have two main uses. The first is for optimization, such as finding the best weights for a neural network.
The second is for supervised feature selection. In this use case, “genes” represent individual features and the “organism” represents a candidate set of features. Each organism in the “population” is graded on a fitness score such as model performance on a hold-out set. The fittest organisms survive and reproduce, repeating until the population converges on a solution some generations later.
- Strengths: Genetic algorithms can efficiently select features from very high dimensional datasets, where exhaustive search is unfeasible. When you need to preprocess data for an algorithm that doesn’t have built-in feature selection (e.g. nearest neighbors) and when you must preserve the original features (i.e. no PCA allowed), GA’s are likely your best bet. These situations can arise in business/client settings that require a transparent and interpretable solution.
- Weaknesses: GA’s add a higher level of complexity to your implementation, and they aren’t worth the hassle in most cases. If possible, it’s faster and simpler to use PCA or to directly use an algorithm with built-in feature selection.
- Implementations: Python / R
4.4. Honorable Mention: Stepwise Search
Stepwise search is a supervised feature selection method based on sequential search, and it has two flavors: forward and backward. For forward stepwise search, you start without any features. Then, you’d train a 1-feature model using each of your candidate features and keep the version with the best performance. You’d continue adding features, one at a time, until your performance improvements stall.
Backward stepwise search is the same process, just reversed: start with all features in your model and then remove one at a time until performance starts to drop substantially.
We note this algorithm purely for historical reasons. Despite many textbooks listing stepwise search as a valid option, it almost always underperforms other supervised methods such as regularization. Stepwise search has many documented flaws, one of the most fatal being that it’s a greedy algorithm that can’t account for future effects of each change. We don’t recommend this method.
5. Feature Extraction
Feature extraction is for creating a new, smaller set of features that stills captures most of the useful information. Again, feature selection keeps a subset of the original features while feature extraction creates new ones.
As with feature selection, some algorithms already have built-in feature extraction. The best example is Deep Learning, which extracts increasingly useful representations of the raw input data through each hidden neural layer. We covered this in Part 1: Modern Machine Learning Algorithms.
As a stand-alone task, feature extraction can be unsupervised (i.e. PCA) or supervised (i.e. LDA).
4.1. Principal Component Analysis (PCA)
Principal component analysis (PCA) is an unsupervised algorithm that creates linear combinations of the original features. The new features are orthogonal, which means that they are uncorrelated. Furthermore, they are ranked in order of their “explained variance.” The first principal component (PC1) explains the most variance in your dataset, PC2 explains the second-most variance, and so on.
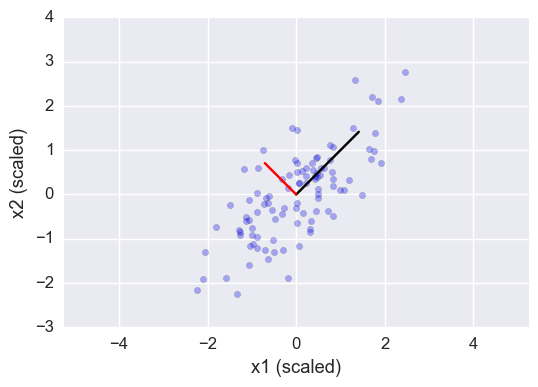
Therefore, you can reduce dimensionality by limiting the number of principal components to keep based on cumulative explained variance. For example, you might decide to keep only as many principal components as needed to reach a cumulative explained variance of 90%.
You should always normalize your dataset before performing PCA because the transformation is dependent on scale. If you don’t, the features that are on the largest scale would dominate your new principal components.
- Strengths: PCA is a versatile technique that works well in practice. It’s fast and simple to implement, which means you can easily test algorithms with and without PCA to compare performance. In addition, PCA offers several variations and extensions (i.e. kernel PCA, sparse PCA, etc.) to tackle specific roadblocks.
- Weaknesses: The new principal components are not interpretable, which may be a deal-breaker in some settings. In addition, you must still manually set or tune a threshold for cumulative explained variance.
- Implementations: Python / R
4.2. Linear Discriminant Analysis (LDA)
Linear discriminant analysis (LDA) – not to be confused with latent Dirichlet allocation – also creates linear combinations of your original features. However, unlike PCA, LDA doesn’t maximize explained variance. Instead, it maximizes the separability between classes.
Therefore, LDA is a supervised method that can only be used with labeled data. So which is better: LDA and PCA? Well, results will vary from problem to problem, and the same “No Free Lunch” theorem from Part 1 applies.
The LDA transformation is also dependent on scale, so you should normalize your dataset first.
- Strengths: LDA is supervised, which can (but doesn’t always) improve the predictive performance of the extracted features. Furthermore, LDA offers variations (i.e. quadratic LDA) to tackle specific roadblocks.
- Weaknesses: As with PCA, the new features are not easily interpretable, and you must still manually set or tune the number of components to keep. LDA also requires labeled data, which makes it more situational.
- Implementations: Python / R
4.3. Autoencoders
Autoencoders are neural networks that are trained to reconstruct their original inputs. For example, image autoencoders are trained to reproduce the original images instead of classifying the image as a dog or a cat.
So how is this helpful? Well, the key is to structure the hidden layer to have fewer neurons than the input/output layers. Thus, that hidden layer will learn to produce a smaller representation of the original image.
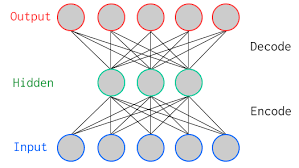
Because you use the input image as the target output, autoencoders are considered unsupervised. They can be used directly (e.g. image compression) or stacked in sequence (e.g. deep learning).
- Strengths: Autoencoders are neural networks, which means they perform well for certain types of data, such as image and audio data.
- Weaknesses: Autoencoders are neural networks, which means they require more data to train. They are not used as general-purpose dimensionality reduction algorithms.
- Implementations: Python / R
Parting Words
We’ve just taken a whirlwind tour through modern algorithms for Dimensionality Reduction, broken into Feature Selection and Feature Extraction.
We’ll leave you with the same parting advice from Part 1: Modern Machine Learning Algorithms.
- Practice, practice, practice. Grab a dataset and strike while the iron is hot.
- Master the fundamentals. For example, it’s more fruitful to first understand the differences between PCA and LDA than to dive into the nuances of LDA versus quadratic-LDA.
- Remember, better data beats fancier algorithms. We repeat this a lot, but it’s the honest darn truth!